animated-bottom-navigation-bar
AnimatedBottomNavigationBar is a customizable widget inspired by dribble shot.
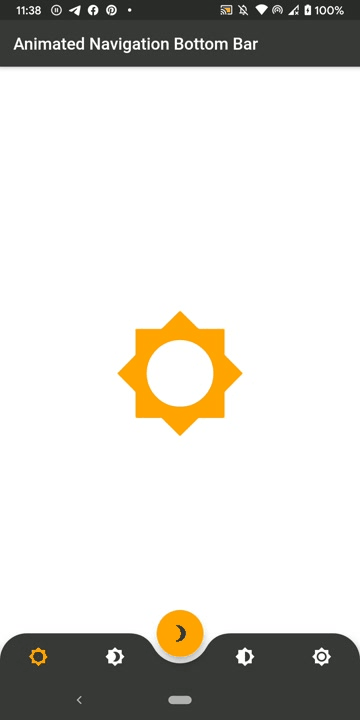
Getting Started
To get started, place your AnimatedBottomNavigationBar
in the bottomNavigationBar slot of a Scaffold
.
The AnimatedBottomNavigationBar
respects FloatingActionButton
location.
For example:
Scaffold(
body: Container(), //destination screen
floatingActionButton: FloatingActionButton(
//params
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
gapLocation: GapLocation.center,
notchSmoothness: NotchSmoothness.verySmoothEdge,
leftCornerRadius: 32,
rightCornerRadius: 32,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Customization
AnimatedBottomNavigationBar is customizable and works with 2, 3, 4, or 5 navigation elements.
Scaffold(
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Scaffold(
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
leftCornerRadius: 32,
rightCornerRadius: 32,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Scaffold(
floatingActionButton: FloatingActionButton(
//params
),
floatingActionButtonLocation: FloatingActionButtonLocation.endDocked,
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
gapLocation: GapLocation.end,
notchSmoothness: NotchSmoothness.defaultEdge,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Scaffold(
floatingActionButton: FloatingActionButton(
//params
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
gapLocation: GapLocation.center,
notchSmoothness: NotchSmoothness.defaultEdge,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Scaffold(
floatingActionButton: FloatingActionButton(
//params
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
gapLocation: GapLocation.center,
notchSmoothness: NotchSmoothness.softEdge,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Scaffold(
floatingActionButton: FloatingActionButton(
//params
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
gapLocation: GapLocation.center,
notchSmoothness: NotchSmoothness.smoothEdge,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Scaffold(
floatingActionButton: FloatingActionButton(
//params
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
gapLocation: GapLocation.center,
notchSmoothness: NotchSmoothness.verySmoothEdge,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Driving Navigation Bar Changes
You have to change the active navigation bar tab programmatically by passing a new activeIndex to the AnimatedBottomNavigationBar widget.
class _MyAppState extends State<MyApp> {
int activeIndex;
/// Handler for when you want to programmatically change
/// the active index. Calling `setState()` here causes
/// Flutter to re-render the tree, which `AnimatedBottomNavigationBar`
/// responds to by running its normal animation.
void _onTap(int index) {
setState((){
activeIndex = index;
});
}
Widget build(BuildContext context) {
return AnimatedBottomNavigationBar(
activeIndex: activeIndex,
onTap: _onTap,
//other params
);
}
}
GitHub
https://github.com/LanarsInc/animated-bottom-navigation-bar-flutter