field_suggestion
Create highly customizable, simple, and controllable autocomplete fields for Flutter.
Additional sources
- Medium Article about FieldSuggestion - Autocomplete fields in Flutter
Installing
See the official installing guidline from - field_suggestion/install
Usage & Overview
Make ready your home widget by creating required options for FieldSuggestion.
final textEditingController = TextEditingController();
// And
List<String> suggestionList = [
'[email protected]',
'[email protected]',
'[email protected]',
];
// Or
List<int> numSuggestions = [
13187829696,
13102743803,
15412917703,
];
// Or
// Note: Take look at [Class suggestions] part.
List<UserModel> userSuggestions = [
UserModel(email: '[email protected]', username: 'user1', password: '1234567'),
UserModel(email: '[email protected]', username: 'user2', password: 'test123'),
UserModel(email: '[email protected]', username: 'user3', password: 'test123')
];
By resolving #31 we can highlight matchers (suggestions).
So, to do that field suggestion includes special made package highlightable.
However, also you can use it without field suggestion.
See the additional sources part and official repo of highlightable to learn how to use it in field suggestion and also without field suggestion
Basic usage.
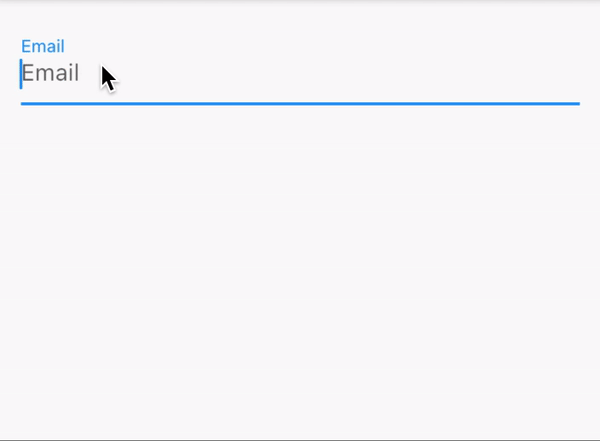
FieldSuggestion(
textController: textEditingController,
suggestionList: suggestionList,
hint: 'Email',
),
Custom usage.
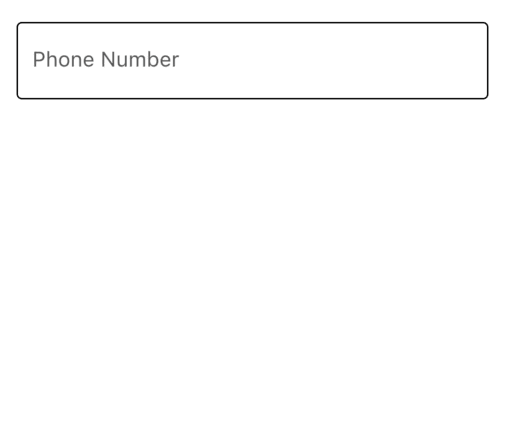
FieldSuggestion(
textController: secondTextController,
suggestionList: numSuggestions,
boxController: secondBoxController,
onItemSelected: (value) {
// Do Something...
},
fieldDecoration: InputDecoration(
hintText: "Phone Number",
enabledBorder: const OutlineInputBorder(),
focusedBorder: const OutlineInputBorder(),
),
wDivider: true,
divider: const SizedBox(height: 5),
wSlideAnimation: true,
slideAnimationStyle: SlideAnimationStyle.LTR,
slideCurve: Curves.linearToEaseOut,
animationDuration: const Duration(milliseconds: 300),
itemStyle: SuggestionItemStyle(
leading: const Icon(Icons.person),
borderRadius: const BorderRadius.all(Radius.circular(5)),
boxShadow: [
const BoxShadow(
blurRadius: 1,
spreadRadius: 1,
offset: Offset(0, 2),
color: Color(0xffD5D5D5),
),
],
),
disableItemTrailing: true,
boxStyle: SuggestionBoxStyle(
backgroundColor: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow: [
BoxShadow(
color: Colors.blue.withOpacity(.2),
spreadRadius: 5,
blurRadius: 10,
offset: const Offset(0, 5),
),
],
),
),
Builder
Also you can create your own suggestion items by using FieldSuggestion.builder()
.
Require to take suggestionList
, textController
, and itemBuilder
.
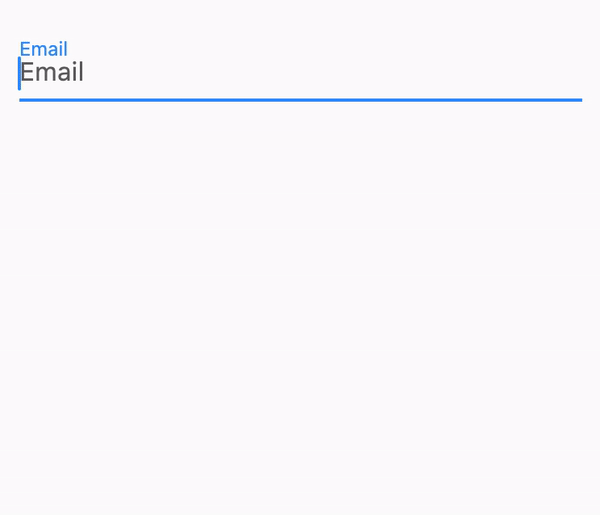
class BuilderExample extends StatelessWidget {
final textEditingController = TextEditingController();
List<String> suggestionsList = ['[email protected]', '[email protected]'];
@override
Widget build(BuildContext context) {
return Scaffold(
body: FieldSuggestion.builder(
hint: 'Email',
textController: textEditingController,
suggestionList: suggestionsList,
itemBuilder: (BuildContext context, int index) {
return GestureDetector(
onTap: () => textEditingController.text = suggestionsList[index],
child: Card(
child: ListTile(
title: Text(suggestionsList[index]),
leading: Container(
height: 30,
width: 30,
decoration: BoxDecoration(
color: Colors.blueGrey,
shape: BoxShape.circle,
),
child: Center(
child: Text(suggestionsList[index][0].toUpperCase()),
),
),
),
),
);
},
),
);
}
}
External control
Here we just wrapped our Scaffold
with GestureDetector
to handle gestures on the screen.
And now we can close box when we tap on the screen. (You can do it everywhere, where you used FieldSuggestion
with BoxController
).
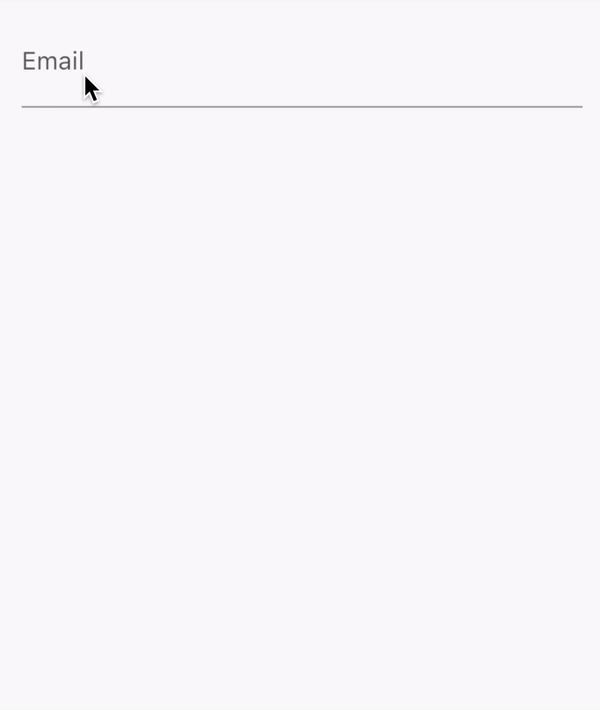
class Example extends StatelessWidget {
final _textController = TextEditingController();
final _boxController = BoxController();
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () => _boxController.close(),
child: Scaffold(
body: Center(
child: FieldSuggestion(
hint: 'test',
suggestionList: [], // Your suggestions list here...
boxController: _boxController,
textController: _textController,
),
),
),
);
}
}
Class suggestions
UserModel
class, we would use it into suggestionList
.
Note: You must have toJson
method in your model class.
class UserModel {
final String? email;
final String? username;
final String? password;
const UserModel({this.email, this.username, this.password});
// If we wanna use this model class into FieldSuggestion,
// then we must to have toJson method. Like that:
Map<String, dynamic> toJson() => {
'email': this.email,
'username': this.username,
'password': this.password,
};
}
If we gave a userSuggestions
which is List<UserModel>
.
Then we must add the searchBy
property. Otherwise we will get an error like:
If given suggestionList's runtimeType isn't List<String>, List<int> or List<double>. That means, you was gave a List which includes dart classes. So then [searchBy] can't be null.
Our model has email, username, and password, right? So then we can implement it like:
searchBy: ['email']
or searchBy: ['email', 'username']
.
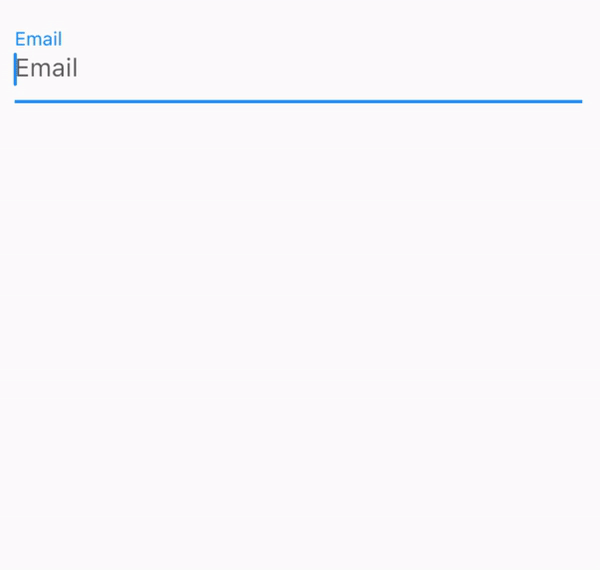
FieldSuggestion(
hint: 'Email',
// If y're using list where are classes,
// Don't forget adding search by property.
searchBy: ['email', 'username'],
itemTitleBy: 'username',
// If you provide [itemSubtitleBy] then suggestion
// item's subtitle automaticallty will be enabled.
itemSubtitleBy: 'email',
boxController: thirdBoxController,
textController: thirdTextController,
suggestionList: userSuggestions,
onItemSelected: (value) {
// The field suggestion needs toJson mehtod inside your model right?
// So that's mean it converts your model to json.
// Then the output has to be JSON (Map). So now we can get our value's email.
print(value['passoword']);
},
),