flutter_shine.dart
Flutter Shine is a library for pretty shadows, dynamic light positions, extremely customizable shadows, no library dependencies, text or box shadows based on content.
Flutter widget inspired by Shine
Installation
Add the Package
dependencies:
flutter_shine: ^0.0.5
❔ Usage
Import this class
import 'package:flutter_shine/flutter_shine.dart';
Flutter Shine
See how easy it is to create a shadow on text and on a container.
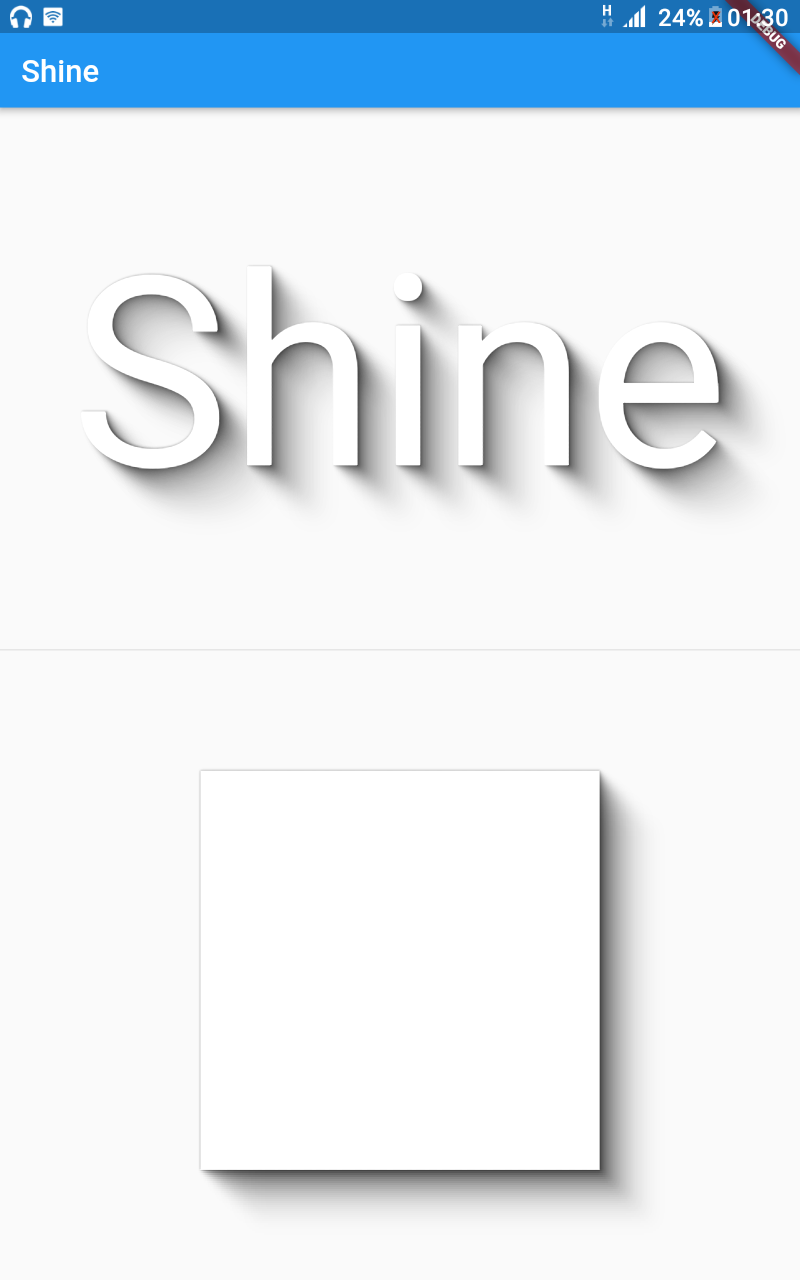
FlutterShine(
builder: (BuildContext context, ShineShadow shineShadow) {
return Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text(
"Shine",
style: TextStyle(
fontSize: 100,
color: Colors.white,
shadows: shineShadow.shadows),
),
Divider(),
Container(
width: 300,
height: 300,
decoration: BoxDecoration(
color: Colors.white, boxShadow: shineShadow.boxShadows),
)
],
);
},
),
Extremely customizable shadow with a dynamic light positions.
You can customize follows values :
- number of Steps : The density of the shadow
- opacity : The opacity of the shadow
- opacity Power : The opacity power
- offset : The offset of the shadow
- offset Power : The offset power
- blur : The blur of the shadow
- blur Power : The blur power
- shadow Color : The color of the shadow
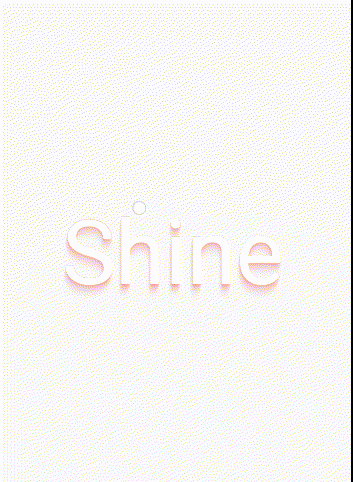
FlutterShine(
[config: Config(shadowColor: Colors.red[300]),]
[light: Light(intensity: 1, position: Point(x, y)),]
builder: (BuildContext context, ShineShadow shineShadow) {
return Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text(
"Shine",
style: TextStyle(
fontSize: 100,
color: Colors.white,
shadows: shineShadow.shadows),
),
Divider(),
Container(
width: 300,
height: 300,
decoration: BoxDecoration(
color: Colors.white, boxShadow: shineShadow.boxShadows),
)
],
);
},
),
Examples
Web and command-line examples can be found in the example
folder.
Web Examples
In order to run the web examples, please follow these steps:
- Clone this repo and enter the directory
- Run
pub get
- Run
pub run build_runner serve example
- Navigate to http://localhost:8080/web/ in your browser
Command Line Examples
In order to run the command line example, please follow these steps:
- Clone this repo and enter the directory
- Run
pub get
- Run
dart example/lib/main.dart
Flutter Example
Install Flutter
In order to run the flutter example, you must have Flutter installed. For installation instructions, view the online
documentation.
Run the app
- Open up an Android Emulator, the iOS Simulator, or connect an appropriate mobile device for debugging.
- Open up a terminal
cd
into theexample/lib/
directory- Run
flutter doctor
to ensure you have all Flutter dependencies working. - Run
flutter packages get
- Run
flutter run