Utilities for loading and running WASM modules from Dart code
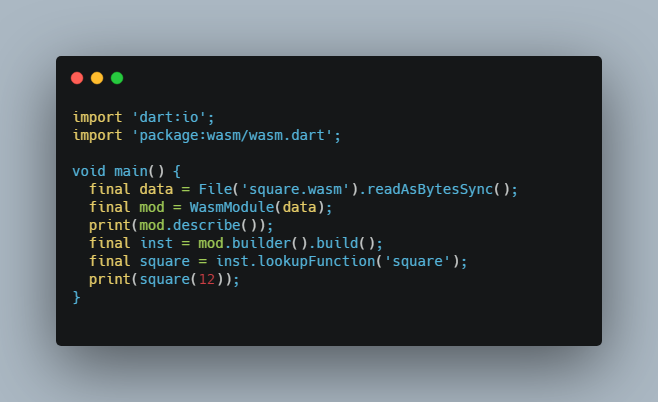
wasm
Provides utilities for loading and running WASM modules
Built on top of the Wasmer runtime.
Setup
Start by installing the tools needed to build the Wasmer runtime:
-
Install the Rust SDK.
-
On Windows, to get
link.exe
, install the Visual Studio build tools
with the "Desktop development with C++"-option selected.
Basic Usage
As a simple example, we'll try to call a simple C function from Dart using
package:wasm
. For a more detailed example that uses WASI, check out the
example directory.
-
First create new Dart app folder:
dart create wasmtest
-
Add a dependency to package
wasm
inpubspec.yaml
and rundart pub get
-
Next run
dart run wasm:setup
to build the Wasmer runtime. This will take a few minutes. -
Then add a new file
square.cc
with the following contents:extern "C" int square(int n) { return n * n; }
-
We can compile this C++ code to WASM using a recent version of the
clang compiler:clang --target=wasm32 -nostdlib "-Wl,--export-all" "-Wl,--no-entry" -o square.wasm square.cc
-
Replace the contents of your Dart program (
bin/wasmtest.dart
) with:import 'dart:io'; import 'package:wasm/wasm.dart'; void main() { final data = File('square.wasm').readAsBytesSync(); final mod = WasmModule(data); print(mod.describe()); final inst = mod.builder().build(); final square = inst.lookupFunction('square'); print(square(12)); }
-
Run the Dart program:
dart run
. This should print:export memory: memory export function: int32 square(int32) 144