Easy way to store http response for flutter
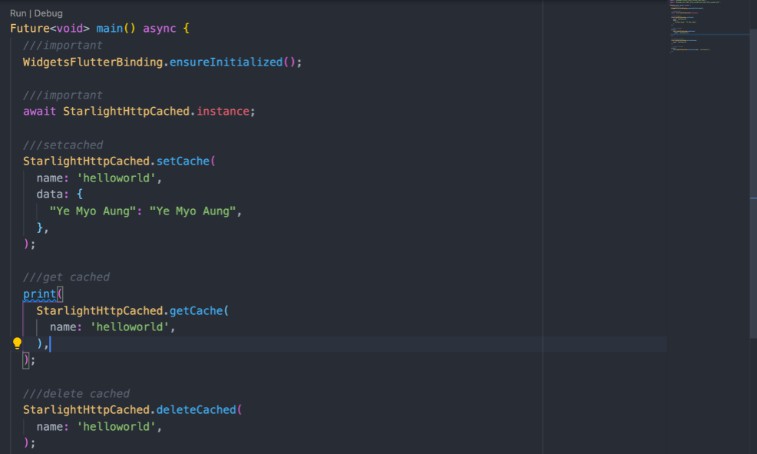
Starlight Http Cached
The easiest way to store data such as http response,String,int,double,bool,map,list.
Features
☑️ Set Cached
☑️ Get Cached
☑️ Delete Cached
☑️ Check Cached
Installation
Add starlight_http_cached as dependency to your pubspec file.
starlight_http_cached:
git:
url: https://github.com/YeMyoAung/starlight_http_cached.git
Usage
First of all you need to import our package.
import 'package:starlight_http_cached/starlight_http_cached.dart';
Initializing StarlightHttpCached
To initialize StarlightHttpCached, call the instance method on the StarlightHttpCached class:
await StarlightHttpCached.instance;
Set Cached
import 'package:flutter/material.dart';
import 'package:starlight_http_cached/starlight_http_cached.dart';
Future<void> main() async {
///important
WidgetsFlutterBinding.ensureInitialized();
///important
await StarlightHttpCached.instance;
///setcached
StarlightHttpCached.setCached(
name: 'helloworld',
data: {
"Ye Myo Aung": "Ye Myo Aung",
},
);
}
Get Cached
import 'package:flutter/material.dart';
import 'package:starlight_http_cached/starlight_http_cached.dart';
Future<void> main() async {
///important
WidgetsFlutterBinding.ensureInitialized();
///important
await StarlightHttpCached.instance;
///get cached
print(
StarlightHttpCached.getCached(
name: 'helloworld',
),
);
}
Delete Cached
import 'package:flutter/material.dart';
import 'package:starlight_http_cached/starlight_http_cached.dart';
Future<void> main() async {
///important
WidgetsFlutterBinding.ensureInitialized();
///important
await StarlightHttpCached.instance;
///delete cached
StarlightHttpCached.deleteCached(
name: 'helloworld',
);
}
Check Cached
import 'package:flutter/material.dart';
import 'package:starlight_http_cached/starlight_http_cached.dart';
Future<void> main() async {
///important
WidgetsFlutterBinding.ensureInitialized();
///important
await StarlightHttpCached.instance;
///check cached
print(
StarlightHttpCached.hasCached(name: 'helloworld'),
);
}